Adding Stripe.js to React
Add to favorites
Loading Stripe.js library to your React client application
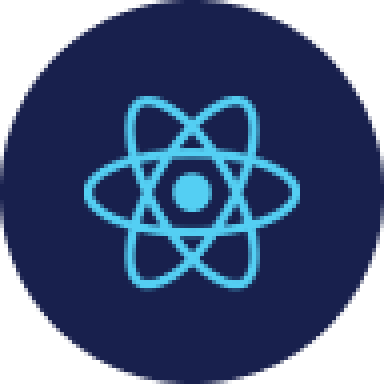
Advanced React Hooks Handbook
1
Intro to Firebase
6:59
2
Firebase Auth
11:59
3
Firestore
10:51
4
Firebase Storage
6:40
5
Serverless Email Sending
10:02
6
Geocoding with Mapbox
9:24
7
Contentful Part 1
4:59
8
Contentful Part 2
8:52
9
Gatsby and Shopify Part 1
5:20
10
Gatsby and Shopify Part 2
13:21
11
Gatsby and Shopify Part 3
14:32
12
Creating Stripe Account and Product
5:18
13
Adding Stripe.js to React
5:54
14
Stripe Checkout Client Only
18:18
15
PayPal Checkout
31:21
To be able to use Stripe Checkout in a client application, Stripe's own library is needed, Stripe.js. This tutorial will go over on how to install that library and load an instance of Stripe. In addition to the basic importing, there is an option to defer instantiating Stripe and activate fraud detection.
Purchase includes access to 50+ courses, 320+ premium tutorials, 300+ hours of videos, source files and certificates.
Learn with videos and source files. Available to Pro subscribers only.
Purchase includes access to 50+ courses, 320+ premium tutorials, 300+ hours of videos, source files and certificates.
Templates and source code
Download source files
Download the videos and assets to refer and learn offline without interuption.
Design template
Source code for all sections
Video files, ePub and subtitles
Browse all downloads
1
Intro to Firebase
Learn about Firebase and set it up in your React project
6:59
2
Firebase Auth
Set up authentication in your React application using Firebase Auth
11:59
3
Firestore
Enable Firestore as your database in your React application
10:51
4
Firebase Storage
Enable Firebase Storage in your application to store photos or videos from users
6:40
5
Serverless Email Sending
Use EmailJS to send an email without backend
10:02
6
Geocoding with Mapbox
Create an address autocomplete with Mapbox's Geocoding API
9:24
7
Contentful Part 1
Create a Contentful account, add a model and content
4:59
8
Contentful Part 2
How to use the Content Delivery API to fetch data from Contentful
8:52
9
Gatsby and Shopify Part 1
Create a Shopify store, generate a password and add products to the store
5:20
10
Gatsby and Shopify Part 2
Connect Shopify to Gatsby and display all products
13:21
11
Gatsby and Shopify Part 3
Create a seamless checkout experience with Shopify and Gatsby
14:32
12
Creating Stripe Account and Product
Start integrating with Stripe and set up an account, product and price
5:18
13
Adding Stripe.js to React
Loading Stripe.js library to your React client application
5:54
14
Stripe Checkout Client Only
Accept payment with Stripe Checkout without backend
18:18
15
PayPal Checkout
Integrate online payment with PayPal
31:21
Meet the instructor
We all try to be consistent with our way of teaching step-by-step, providing source files and prioritizing design in our courses.
Dara To
Full-stack Developer
I'm a former financial analyst turned coder. Vegetarian, health-centered, dog owner.
5 courses - 25 hours
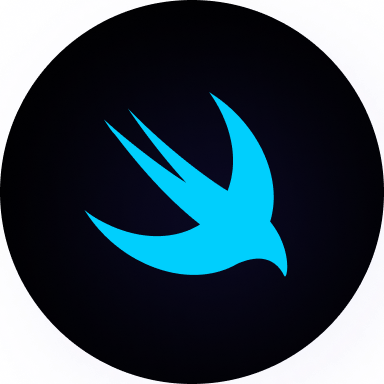
UI and Animations in SwiftUI
Level up your UI and animation skills by implementing various applications from custom designs in SwiftUI
4 hrs
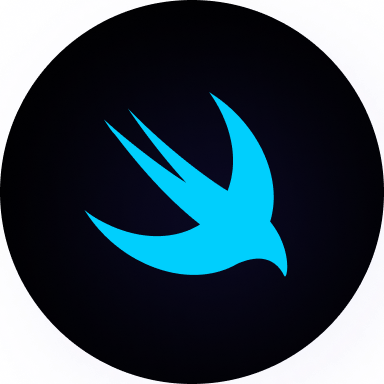
Build an Expense Tracker App in SwiftUI
Design and code a SwiftUI 3 app in Xcode 13 with data modeling, data networking, Combine, MVVM and libraries for custom icons and charts.
3 hrs
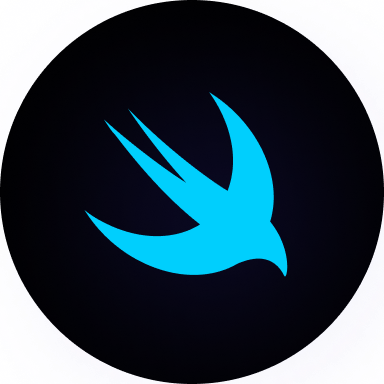
Build Quick Apps with SwiftUI
Apply your Swift and SwiftUI knowledge by building real, quick and various applications from scratch
11 hrs
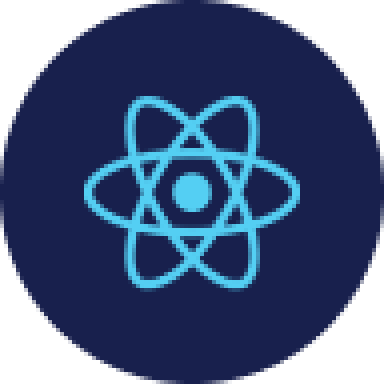
Advanced React Hooks Handbook
An extensive series of tutorials covering advanced topics related to React hooks, with a main focus on backend and logic to take your React skills to the next level
3 hrs
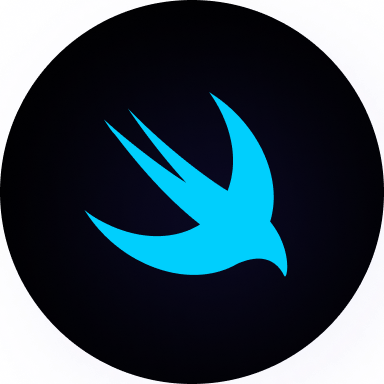
Build an ARKit 2 App
Introduction to ARKit and learn how to make your own playground. You will be able to add models or even your own designs into the app and play with them
4 hrs